C++到C#核心语法对照指南
C++到C#核心语法对照指南
一、基础语法对比
1. 程序入口点
C++:
int main(int argc, char* argv[]) {
std::cout << "Hello World!" << std::endl;
return 0;
}
C#:
// 传统方式
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
// C# 9.0+ 顶级语句
Console.WriteLine("Hello World!");
2. 变量声明与初始化
C++:
// 基本类型
int number = 42;
double pi = 3.14;
bool flag = true;
// 指针
int* ptr = new int(42);
delete ptr;
// 智能指针
std::unique_ptr<int> smartPtr(new int(42));
std::shared_ptr<int> sharedPtr = std::make_shared<int>(42);
// 常量
const int MAX_VALUE = 100;
constexpr int COMPUTED = 10 * 10;
C#:
// 基本类型
int number = 42;
double pi = 3.14;
bool flag = true;
// 引用类型
object obj = new(); // C# 9.0+简化语法
string str = "Hello";
// 常量
const int MaxValue = 100;
readonly int computed = 10 * 10;
// var关键字(类型推断)
var inferred = 42; // 推断为int
var text = "Hello"; // 推断为string
3. 数组声明与使用
C++:
// 固定数组
int arr1[5] = {1, 2, 3, 4, 5};
// 动态数组
int* arr2 = new int[5];
delete[] arr2;
// vector(动态数组)
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.push_back(6);
// 多维数组
int matrix[3][3] = {{1,2,3}, {4,5,6}, {7,8,9}};
C#:
// 一维数组
int[] arr1 = { 1, 2, 3, 4, 5 };
// 动态创建
int[] arr2 = new int[5];
// List(动态列表)
List<int> list = new() { 1, 2, 3, 4, 5 };
list.Add(6);
// 多维数组
int[,] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
// 交错数组
int[][] jaggedArray = new int[3][];
jaggedArray[0] = new int[] { 1, 2, 3 };
4. 字符串处理
C++:
// 字符串声明
const char* str1 = "Hello";
std::string str2 = "World";
// 字符串连接
std::string result = str2 + " " + str1;
// 字符串格式化
char buffer[100];
sprintf(buffer, "Value: %d", 42);
// 使用stringstream
std::stringstream ss;
ss << "Value: " << 42;
std::string formatted = ss.str();
// 字符串分割
std::string input = "a,b,c";
std::stringstream ss(input);
std::string token;
std::vector<std::string> tokens;
while (std::getline(ss, token, ',')) {
tokens.push_back(token);
}
C#:
// 字符串声明
string str1 = "Hello";
string str2 = "World";
// 字符串连接
string result1 = str1 + " " + str2;
string result2 = string.Concat(str1, " ", str2);
string result3 = $"{str1} {str2}"; // 字符串插值
// 字符串格式化
string formatted1 = string.Format("Value: {0}", 42);
string formatted2 = $"Value: {42}";
// 字符串分割
string input = "a,b,c";
string[] tokens = input.Split(',');
// 字符串构建器(高效字符串操作)
StringBuilder sb = new();
sb.Append("Hello");
sb.Append(" World");
string final = sb.ToString();
二、面向对象编程对比
1. 类的定义和继承
C++:
// Animal.h
class Animal {
protected:
std::string name;
public:
Animal(const std::string& n) : name(n) {}
virtual ~Animal() = default;
virtual void MakeSound() = 0;
virtual std::string GetName() const { return name; }
};
// Dog.h
class Dog : public Animal {
private:
int age;
public:
Dog(const std::string& n, int a) : Animal(n), age(a) {}
void MakeSound() override {
std::cout << "Woof!" << std::endl;
}
};
C#:
public abstract class Animal
{
protected string Name { get; set; }
protected Animal(string name)
{
Name = name;
}
public abstract void MakeSound();
public virtual string GetName() => Name;
}
public class Dog : Animal
{
private int age;
public Dog(string name, int age) : base(name)
{
this.age = age;
}
public override void MakeSound()
{
Console.WriteLine("Woof!");
}
}
2. 属性实现
C++:
class Person {
private:
std::string name;
int age;
public:
// Getter和Setter方法
std::string GetName() const { return name; }
void SetName(const std::string& value) { name = value; }
int GetAge() const { return age; }
void SetAge(int value) {
if (value >= 0) age = value;
}
// 只读方法
std::string GetFullInfo() const {
return name + ", " + std::to_string(age) + " years old";
}
};
C#:
public class Person
{
// 自动属性
public string Name { get; set; }
// 带验证的完整属性
private int age;
public int Age
{
get => age;
set => age = value >= 0 ? value : throw new ArgumentException("Age cannot be negative");
}
// 只读属性
public string FullInfo => $"{Name}, {Age} years old";
// 计算属性
public bool IsAdult => Age >= 18;
// 带后备字段的属性
private string email;
public string Email
{
get => email;
set => email = value?.ToLower();
}
}
3. 接口实现
C++:
// 使用抽象类模拟接口
class IMovable {
public:
virtual ~IMovable() = default;
virtual void Move() = 0;
virtual double GetSpeed() = 0;
virtual void SetSpeed(double speed) = 0;
};
class IDrawable {
public:
virtual ~IDrawable() = default;
virtual void Draw() = 0;
};
// 实现多个接口
class Car : public IMovable, public IDrawable {
private:
double speed;
public:
void Move() override {
std::cout << "Moving at " << speed << std::endl;
}
double GetSpeed() override { return speed; }
void SetSpeed(double s) override { speed = s; }
void Draw() override {
std::cout << "Drawing car" << std::endl;
}
};
C#:
public interface IMovable
{
void Move();
double Speed { get; set; }
}
public interface IDrawable
{
void Draw();
}
// 实现多个接口
public class Car : IMovable, IDrawable
{
public double Speed { get; set; }
public void Move()
{
Console.WriteLine($"Moving at {Speed}");
}
public void Draw()
{
Console.WriteLine("Drawing car");
}
}
// C# 8.0+ 接口默认实现
public interface ILogger
{
void Log(string message) => Console.WriteLine(message);
void LogError(string message) => Console.WriteLine($"Error: {message}");
}
4. 泛型/模板对比
C++:
// 模板类
template<typename T>
class Container {
private:
T data;
public:
void SetData(const T& value) { data = value; }
T GetData() const { return data; }
};
// 模板函数
template<typename T>
T Max(T a, T b) {
return (a > b) ? a : b;
}
// 模板特化
template<>
class Container<bool> {
private:
bool data;
public:
void SetData(bool value) { data = value; }
bool GetData() const { return data; }
};
// 模板约束(C++20 concepts)
template<typename T>
requires std::integral<T>
T Add(T a, T b) {
return a + b;
}
C#:
// 泛型类
public class Container<T>
{
private T data;
public T Data
{
get => data;
set => data = value;
}
}
// 泛型方法
public T Max<T>(T a, T b) where T : IComparable<T>
{
return a.CompareTo(b) > 0 ? a : b;
}
// 多重约束
public class Repository<T> where T : class, IEntity, new()
{
public T CreateNew() => new T();
}
// 泛型接口
public interface IRepository<T> where T : class
{
T GetById(int id);
void Save(T entity);
}
// 协变和逆变
public interface IReader<out T> // 协变
{
T Read();
}
public interface IWriter<in T> // 逆变
{
void Write(T value);
}
三、高级特性对比
1. 异常处理
C++:
class DatabaseException : public std::exception {
public:
const char* what() const noexcept override {
return "Database error occurred";
}
};
void ProcessData() {
try {
// 可能抛出异常的代码
throw DatabaseException();
// 多个异常
throw std::runtime_error("Other error");
}
catch (const DatabaseException& e) {
std::cerr << "Database error: " << e.what() << std::endl;
throw; // 重新抛出
}
catch (const std::exception& e) {
std::cerr << "Standard exception: " << e.what() << std::endl;
}
catch (...) {
std::cerr << "Unknown exception" << std::endl;
}
}
C#:
public class DatabaseException : Exception
{
public DatabaseException(string message) : base(message) { }
public DatabaseException(string message, Exception inner)
: base(message, inner) { }
}
public void ProcessData()
{
try
{
// 可能抛出异常的代码
throw new DatabaseException("Connection failed");
}
catch (DatabaseException ex) when (ex.Message.Contains("Connection"))
{
// 条件捕获(C# 6.0+)
Logger.LogError(ex);
throw; // 保留堆栈跟踪重新抛出
}
catch (Exception ex)
{
Logger.LogError(ex);
// 包装异常
throw new ApplicationException("Processing failed", ex);
}
finally
{
// 清理代码,总是执行
CleanupResources();
}
}
2. 委托和事件
C++:
// 使用函数指针
typedef void (*CallbackFunc)(const std::string&);
class EventEmitter {
private:
CallbackFunc callback;
public:
void SetCallback(CallbackFunc cb) {
callback = cb;
}
void Emit(const std::string& message) {
if (callback) callback(message);
}
};
// 使用std::function
class ModernEmitter {
private:
std::function<void(const std::string&)> callback;
public:
void SetCallback(const std::function<void(const std::string&)>& cb) {
callback = cb;
}
void Emit(const std::string& message) {
if (callback) callback(message);
}
};
C#:
// 委托定义
public delegate void MessageHandler(string message);
public class EventEmitter
{
// 事件声明
public event MessageHandler MessageReceived;
// 事件触发
protected virtual void OnMessageReceived(string message)
{
MessageReceived?.Invoke(message);
}
// 内置委托类型
public Action<string> Logger { get; set; }
public Func<int, int, int> Calculator { get; set; }
public void ProcessMessage(string msg)
{
// 触发事件
OnMessageReceived(msg);
// 使用委托
Logger?.Invoke(msg);
int result = Calculator?.Invoke(5, 3) ?? 0;
}
}
// 使用示例
public void SetupEvents()
{
var emitter = new EventEmitter();
// 订阅事件
emitter.MessageReceived += HandleMessage;
// Lambda表达式
emitter.MessageReceived += (msg) => Console.WriteLine(msg);
// 委托赋值
emitter.Logger = Console.WriteLine;
emitter.Calculator = (x, y) => x + y;
}
3. 异步编程
C++:
// 使用std::future和std::async
#include <future>
std::future<int> AsyncOperation() {
return std::async(std::launch::async, []() {
std::this_thread::sleep_for(std::chrono::seconds(1));
return 42;
});
}
void ProcessAsync() {
auto future = AsyncOperation();
// ... 做其他工作 ...
int result = future.get(); // 等待结果
}
// 使用Promise
void ComplexAsyncOperation() {
std::promise<int> promise;
std::future<int> future = promise.get_future();
std::thread([&promise]() {
try {
int result = PerformWork();
promise.set_value(result);
}
catch (...) {
promise.set_exception(std::current_exception());
}
}).detach();
// ... 等待结果 ...
try {
int value = future.get();
}
catch (const std::exception& e) {
// 处理错误
}
}
C#:
public class AsyncExample
{
// 异步方法
public async Task<int> GetDataAsync()
{
await Task.Delay(1000); // 模拟异步操作
return 42;
}
// 异步流
public async IAsyncEnumerable<int> GenerateNumbersAsync()
{
for (int i = 0; i < 10; i++)
{
await Task.Delay(100);
yield return i;
}
}
// 并行处理
public async Task ProcessItemsAsync(IEnumerable<string> items)
{
// 并行异步操作
var tasks = items.Select(async item =>
{
await Task.Delay(100);
return await ProcessItemAsync(item);
});
// 等待所有任务完成
var results = await Task.WhenAll(tasks);
}
// 异步异常处理
public async Task SafeOperationAsync()
{
try
{
await Task.Delay(100);
throw new Exception("Async error");
}
catch (Exception ex)
{
await LogErrorAsync(ex);
throw;
}
}
// 取消操作
public async Task CancellableOperationAsync(
CancellationToken cancellationToken)
{
while (!cancellationToken.IsCancellationRequested)
{
await Task.Delay(100, cancellationToken);
// 执行工作
}
}
}
// 使用示例
public async Task Example()
{
var asyncExample = new AsyncExample();
// 等待单个任务
int result = await asyncExample.GetDataAsync();
// 处理异步流
await foreach (var number in asyncExample.GenerateNumbersAsync())
{
Console.WriteLine(number);
}
// 使用取消令牌
using var cts = new CancellationTokenSource();
cts.CancelAfter(TimeSpan.FromSeconds(5)); // 5秒后取消
try
{
await asyncExample.CancellableOperationAsync(cts.Token);
}
catch (OperationCanceledException)
{
Console.WriteLine("Operation was cancelled");
}
}
四、集合操作和LINQ对比
C++:
// STL容器操作
vector<int> numbers = {1, 2, 3, 4, 5};
// 过滤
vector<int> filtered;
copy_if(numbers.begin(), numbers.end(),
back_inserter(filtered),
[](int n) { return n % 2 == 0; });
// 转换
vector<string> strings;
transform(numbers.begin(), numbers.end(),
back_inserter(strings),
[](int n) { return std::to_string(n); });
// 排序
sort(numbers.begin(), numbers.end());
sort(numbers.begin(), numbers.end(),
[](int a, int b) { return a > b; });
// 聚合
int sum = accumulate(numbers.begin(), numbers.end(), 0);
auto maxElement = max_element(numbers.begin(), numbers.end());
// 查找
auto it = find(numbers.begin(), numbers.end(), 3);
bool exists = it != numbers.end();
C#:
// LINQ操作
var numbers = new List<int> { 1, 2, 3, 4, 5 };
// 过滤
var filtered = numbers.Where(n => n % 2 == 0);
// 转换
var strings = numbers.Select(n => n.ToString());
// 排序
var ordered = numbers.OrderBy(n => n);
var descending = numbers.OrderByDescending(n => n);
// 聚合
var sum = numbers.Sum();
var max = numbers.Max();
var avg = numbers.Average();
// 复杂查询
var result = numbers
.Where(n => n > 2)
.OrderBy(n => n)
.Select(n => new { Number = n, Square = n * n })
.ToList();
// 分组
var groups = numbers
.GroupBy(n => n % 2)
.Select(g => new {
Key = g.Key,
Items = g.ToList()
});
// 连接
var list1 = new List<(int Id, string Name)>
{ (1, "John"), (2, "Jane") };
var list2 = new List<(int Id, int Score)>
{ (1, 100), (2, 95) };
var joined = list1.Join(
list2,
l1 => l1.Id,
l2 => l2.Id,
(l1, l2) => new {
l1.Name,
l2.Score
});
2. 文件操作
C++:
// 文件写入
void WriteFile() {
ofstream file("data.txt");
if (file.is_open()) {
file << "Hello World" << endl;
file.close();
}
}
// 文件读取
void ReadFile() {
ifstream file("data.txt");
string line;
if (file.is_open()) {
while (getline(file, line)) {
cout << line << endl;
}
file.close();
}
}
// 二进制文件操作
void BinaryFileOps() {
// 写入
ofstream file("data.bin", ios::binary);
int data = 42;
file.write(reinterpret_cast<char*>(&data), sizeof(data));
file.close();
// 读取
ifstream inFile("data.bin", ios::binary);
inFile.read(reinterpret_cast<char*>(&data), sizeof(data));
inFile.close();
}
C#:
public class FileOperations
{
// 同步文件操作
public void WriteFile()
{
File.WriteAllText("data.txt", "Hello World");
// 使用StreamWriter
using var writer = new StreamWriter("data.txt");
writer.WriteLine("Hello World");
}
// 异步文件操作
public async Task WriteFileAsync()
{
await File.WriteAllTextAsync("data.txt", "Hello World");
// 使用StreamWriter
await using var writer = new StreamWriter("data.txt");
await writer.WriteLineAsync("Hello World");
}
// 文件读取
public async Task<string> ReadFileAsync()
{
// 简单读取
string content = await File.ReadAllTextAsync("data.txt");
// 流式读取
await using var stream = File.OpenRead("data.txt");
using var reader = new StreamReader(stream);
string? line;
while ((line = await reader.ReadLineAsync()) != null)
{
Console.WriteLine(line);
}
return content;
}
// 文件监控
public void WatchFile()
{
var watcher = new FileSystemWatcher(".");
watcher.Changed += (s, e) =>
Console.WriteLine($"File {e.Name} changed");
watcher.EnableRaisingEvents = true;
}
// 文件操作异常处理
public async Task SafeFileOperationAsync()
{
try
{
await File.WriteAllTextAsync("data.txt", "Hello World");
}
catch (IOException ex)
{
Console.WriteLine($"IO Error: {ex.Message}");
}
catch (UnauthorizedAccessException ex)
{
Console.WriteLine($"Access Error: {ex.Message}");
}
}
}
3. 网络操作
C++:
// 使用Boost.Asio或原生Socket
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
void NetworkOperation() {
try {
boost::asio::io_context io_context;
tcp::socket socket(io_context);
tcp::resolver resolver(io_context);
auto endpoints = resolver.resolve("example.com", "80");
boost::asio::connect(socket, endpoints);
boost::asio::write(socket,
boost::asio::buffer("GET / HTTP/1.1\r\n\r\n"));
char data[1024];
size_t length = socket.read_some(
boost::asio::buffer(data, 1024));
}
catch (std::exception& e) {
std::cerr << e.what() << std::endl;
}
}
C#:
public class NetworkOperations
{
private readonly HttpClient _client = new();
// HTTP GET请求
public async Task<string> GetDataAsync(string url)
{
try
{
var response = await _client.GetAsync(url);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
catch (HttpRequestException ex)
{
Console.WriteLine($"HTTP Error: {ex.Message}");
throw;
}
}
// HTTP POST请求
public async Task<string> PostDataAsync(
string url, object data)
{
var content = new StringContent(
JsonSerializer.Serialize(data),
Encoding.UTF8,
"application/json");
var response = await _client.PostAsync(url, content);
return await response.Content.ReadAsStringAsync();
}
// WebSocket操作
public async Task WebSocketOperationAsync()
{
using var ws = new ClientWebSocket();
await ws.ConnectAsync(
new Uri("ws://example.com"),
CancellationToken.None);
var buffer = new byte[1024];
while (ws.State == WebSocketState.Open)
{
var result = await ws.ReceiveAsync(
new ArraySegment<byte>(buffer),
CancellationToken.None);
if (result.MessageType == WebSocketMessageType.Close)
{
await ws.CloseAsync(
WebSocketCloseStatus.NormalClosure,
string.Empty,
CancellationToken.None);
}
else
{
// 处理接收到的数据
}
}
}
}
五、并发编程对比
1. 线程操作
C++:
#include <thread>
#include <mutex>
class ThreadExample {
private:
std::mutex mtx;
public:
// 基本线程创建
void BasicThread() {
std::thread t([]() {
std::cout << "Thread running" << std::endl;
});
t.join(); // 等待线程完成
}
// 互斥锁
void MutexExample() {
std::lock_guard<std::mutex> lock(mtx);
// 临界区代码
}
// 条件变量
void ConditionVariableExample() {
std::condition_variable cv;
std::mutex mutex;
bool ready = false;
std::thread worker([&]() {
std::unique_lock<std::mutex> lock(mutex);
cv.wait(lock, [&]() { return ready; });
// 执行工作
});
{
std::lock_guard<std::mutex> lock(mutex);
ready = true;
}
cv.notify_one();
worker.join();
}
};
C#:
public class ThreadExample
{
private readonly object _lock = new();
// 基本线程创建
public void BasicThread()
{
var thread = new Thread(() =>
{
Console.WriteLine("Thread running");
});
thread.Start();
thread.Join(); // 等待线程完成
}
// 线程池使用
public void ThreadPoolExample()
{
ThreadPool.QueueUserWorkItem(state =>
{
Console.WriteLine("Work item running");
});
}
// 锁定示例
public void LockExample()
{
lock (_lock)
{
// 临界区代码
}
}
// Monitor使用
public void MonitorExample()
{
Monitor.Enter(_lock);
try
{
// 临界区代码
}
finally
{
Monitor.Exit(_lock);
}
}
// 异步等待
public async Task WaitExample()
{
using var semaphore = new SemaphoreSlim(1);
await semaphore.WaitAsync();
try
{
// 临界区代码
}
finally
{
semaphore.Release();
}
}
}
2. 并发集合
C++:
#include <concurrent_queue>
#include <concurrent_vector>
class ConcurrentCollections {
public:
void QueueExample() {
concurrency::concurrent_queue<int> queue;
// 添加元素
queue.push(42);
// 尝试获取元素
int value;
if (queue.try_pop(value)) {
// 处理值
}
}
void VectorExample() {
concurrency::concurrent_vector<int> vec;
// 并发添加元素
vec.push_back(1);
// 并发访问
for (const auto& item : vec) {
// 处理元素
}
}
};
C#:
public class ConcurrentCollections
{
// 并发队列
public async Task ConcurrentQueueExample()
{
var queue = new ConcurrentQueue<int>();
// 添加元素
queue.Enqueue(42);
// 尝试获取元素
if (queue.TryDequeue(out int result))
{
Console.WriteLine(result);
}
// 并行操作
await Parallel.ForEachAsync(
Enumerable.Range(0, 100),
async (i, token) =>
{
queue.Enqueue(i);
await Task.Delay(10, token);
});
}
// 并发字典
public void ConcurrentDictionaryExample()
{
var dict = new ConcurrentDictionary<string, int>();
// 添加或更新
dict.AddOrUpdate(
"key",
1, // 添加的值
(key, oldValue) => oldValue + 1 // 更新的值
);
// 获取或添加
var value = dict.GetOrAdd("key", 0);
// 线程安全的更新
dict.AddOrUpdate(
"counter",
1,
(key, old) => old + 1);
}
// 并发包
public void ConcurrentBagExample()
{
var bag = new ConcurrentBag<int>();
// 添加元素
bag.Add(42);
// 尝试获取元素
if (bag.TryTake(out int item))
{
Console.WriteLine(item);
}
}
}
3. 任务并行库(TPL)
C++:
#include <execution>
#include <algorithm>
class ParallelProcessing {
public:
void ParallelAlgorithms() {
std::vector<int> vec(1000);
// 并行填充
std::fill(std::execution::par,
vec.begin(), vec.end(), 42);
// 并行排序
std::sort(std::execution::par,
vec.begin(), vec.end());
// 并行转换
std::transform(std::execution::par,
vec.begin(), vec.end(),
vec.begin(),
[](int x) { return x * 2; });
}
};
C#:
public class ParallelProcessing
{
// 并行For循环
public void ParallelForExample()
{
Parallel.For(0, 1000, i =>
{
// 并行处理每个索引
ProcessItem(i);
});
}
// 并行ForEach
public void ParallelForEachExample()
{
var items = Enumerable.Range(0, 1000);
Parallel.ForEach(
items,
new ParallelOptions { MaxDegreeOfParallelism = 4 },
item =>
{
// 并行处理每个项
ProcessItem(item);
});
}
// 并行LINQ
public void ParallelLinqExample()
{
var numbers = Enumerable.Range(0, 1000);
var result = numbers.AsParallel()
.Where(n => n % 2 == 0)
.Select(n => n * 2)
.OrderBy(n => n)
.ToList();
}
// 任务并行
public async Task ParallelTasksExample()
{
var tasks = new List<Task<int>>();
// 创建多个任务
for (int i = 0; i < 10; i++)
{
tasks.Add(Task.Run(async () =>
{
await Task.Delay(100);
return await ProcessItemAsync(i);
}));
}
// 等待所有任务完成
var results = await Task.WhenAll(tasks);
// 获取第一个完成的任务
var firstResult = await Task.WhenAny(tasks);
}
}
六、反射和元数据编程
1. 反射基础
C++:
// C++没有内置的反射机制,通常使用自定义宏或第三方库
#include <typeinfo>
class ReflectionExample {
public:
// 使用RTTI (Run-Time Type Information)
void TypeInfo() {
int x = 42;
const std::type_info& typeInfo = typeid(x);
std::cout << typeInfo.name() << std::endl;
}
};
// 模拟反射的一种方式
#define REGISTER_PROPERTY(Type, Name) \
Type Name; \
const char* Get##Name() { return #Name; }
class Person {
REGISTER_PROPERTY(std::string, name)
REGISTER_PROPERTY(int, age)
};
C#:
public class ReflectionExample
{
public void TypeInformation()
{
// 获取类型信息
Type type = typeof(string);
Type type2 = "Hello".GetType();
// 检查类型信息
Console.WriteLine($"Type: {type.Name}");
Console.WriteLine($"Is class: {type.IsClass}");
Console.WriteLine($"Base type: {type.BaseType?.Name}");
}
public void MemberInformation()
{
Type type = typeof(Person);
// 获取所有公共属性
var properties = type.GetProperties();
// 获取所有公共方法
var methods = type.GetMethods();
// 获取特定成员
var property = type.GetProperty("Name");
var method = type.GetMethod("ToString");
// 获取特性
var attributes = type.GetCustomAttributes();
}
public void DynamicInstantiation()
{
// 动态创建实例
Type type = typeof(Person);
object instance = Activator.CreateInstance(type);
// 动态调用方法
var method = type.GetMethod("SayHello");
method?.Invoke(instance, null);
// 动态设置属性
var property = type.GetProperty("Name");
property?.SetValue(instance, "John");
}
}
// 使用特性的示例
[Serializable]
public class Person
{
[Required]
public string Name { get; set; }
[Range(0, 150)]
public int Age { get; set; }
public void SayHello()
{
Console.WriteLine($"Hello, I'm {Name}");
}
}
2. 序列化
C++:
// 使用Boost.Serialization或自定义序列化
#include <boost/archive/text_oarchive.hpp>
#include <boost/archive/text_iarchive.hpp>
class SerializableClass {
private:
std::string name;
int age;
friend class boost::serialization::access;
template<class Archive>
void serialize(Archive & ar, const unsigned int version)
{
ar & name;
ar & age;
}
public:
void Save() {
std::ofstream file("data.txt");
boost::archive::text_oarchive oa(file);
oa << *this;
}
void Load() {
std::ifstream file("data.txt");
boost::archive::text_iarchive ia(file);
ia >> *this;
}
};
C#:
public class SerializationExample
{
// JSON序列化
public string JsonSerialize<T>(T obj)
{
return JsonSerializer.Serialize(obj, new JsonSerializerOptions
{
WriteIndented = true,
PropertyNamingPolicy = JsonNamingPolicy.CamelCase
});
}
public T JsonDeserialize<T>(string json)
{
return JsonSerializer.Deserialize<T>(json);
}
// XML序列化
public string XmlSerialize<T>(T obj)
{
var serializer = new XmlSerializer(typeof(T));
using var writer = new StringWriter();
serializer.Serialize(writer, obj);
return writer.ToString();
}
public T XmlDeserialize<T>(string xml)
{
var serializer = new XmlSerializer(typeof(T));
using var reader = new StringReader(xml);
return (T)serializer.Deserialize(reader);
}
// 二进制序列化
public byte[] BinarySerialize<T>(T obj)
{
using var stream = new MemoryStream();
var formatter = new BinaryFormatter();
formatter.Serialize(stream, obj);
return stream.ToArray();
}
public T BinaryDeserialize<T>(byte[] data)
{
using var stream = new MemoryStream(data);
var formatter = new BinaryFormatter();
return (T)formatter.Deserialize(stream);
}
}
// 序列化示例类
[Serializable]
public class Person
{
[JsonPropertyName("fullName")]
public string Name { get; set; }
[JsonIgnore]
public int InternalId { get; set; }
[XmlElement("DateOfBirth")]
public DateTime BirthDate { get; set; }
}
3. 依赖注入
C++:
// C++通常使用工厂模式或依赖注入容器
class ILogger {
public:
virtual ~ILogger() = default;
virtual void Log(const std::string& message) = 0;
};
class ConsoleLogger : public ILogger {
public:
void Log(const std::string& message) override {
std::cout << message << std::endl;
}
};
class Service {
private:
std::shared_ptr<ILogger> logger;
public:
Service(std::shared_ptr<ILogger> logger)
: logger(logger) {}
void DoWork() {
logger->Log("Working...");
}
};
// 使用示例
auto logger = std::make_shared<ConsoleLogger>();
Service service(logger);
C#:
// 使用内置依赖注入
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
// 注册服务
services.AddSingleton<ILogger, ConsoleLogger>();
services.AddScoped<IUserService, UserService>();
services.AddTransient<IEmailSender, SmtpEmailSender>();
// 注册带配置的服务
services.Configure<EmailSettings>(configuration.GetSection("Email"));
// 注册工厂
services.AddSingleton<IDatabase>(sp =>
{
var config = sp.GetRequiredService<IConfiguration>();
return new Database(config.GetConnectionString("Default"));
});
}
}
// 服务类
public interface IUserService
{
Task<User> GetUserAsync(int id);
}
public class UserService : IUserService
{
private readonly ILogger<UserService> _logger;
private readonly IEmailSender _emailSender;
// 构造函数注入
public UserService(
ILogger<UserService> logger,
IEmailSender emailSender)
{
_logger = logger;
_emailSender = emailSender;
}
public async Task<User> GetUserAsync(int id)
{
_logger.LogInformation($"Getting user {id}");
// 实现逻辑
return await Task.FromResult(new User());
}
}
// 控制器示例
public class UserController : ControllerBase
{
private readonly IUserService _userService;
public UserController(IUserService userService)
{
_userService = userService;
}
[HttpGet("{id}")]
public async Task<ActionResult<User>> GetUser(int id)
{
return await _userService.GetUserAsync(id);
}
}
七、性能优化和内存管理
1. 内存管理
C++:
class MemoryManagement {
public:
// 手动内存管理
void RawPointers() {
// 堆分配
int* ptr = new int(42);
delete ptr; // 必须手动释放
// 数组
int* arr = new int[100];
delete[] arr;
}
// 智能指针
void SmartPointers() {
// 独占所有权
std::unique_ptr<int> unique(new int(42));
// 共享所有权
std::shared_ptr<int> shared = std::make_shared<int>(42);
// 弱引用
std::weak_ptr<int> weak = shared;
}
// RAII模式
class ResourceHandler {
FILE* file;
public:
ResourceHandler(const char* filename) {
file = fopen(filename, "r");
}
~ResourceHandler() {
if (file) fclose(file);
}
};
};
C#:
public class MemoryManagement
{
// 托管资源
public class ManagedResources
{
private readonly List<byte[]> _cache = new();
public void AllocateMemory()
{
// GC会自动管理这些对象
var data = new byte[1024];
_cache.Add(data);
}
}
// 非托管资源管理
public class UnmanagedResources : IDisposable
{
private bool _disposed;
private IntPtr _nativeResource;
private readonly SafeHandle _managedResource;
public UnmanagedResources()
{
_nativeResource = Marshal.AllocHGlobal(100);
_managedResource = new SafeFileHandle(IntPtr.Zero, true);
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!_disposed)
{
if (disposing)
{
// 释放托管资源
_managedResource?.Dispose();
}
// 释放非托管资源
if (_nativeResource != IntPtr.Zero)
{
Marshal.FreeHGlobal(_nativeResource);
_nativeResource = IntPtr.Zero;
}
_disposed = true;
}
}
~UnmanagedResources()
{
Dispose(false);
}
}
// 内存优化技巧
public class MemoryOptimization
{
// 使用对象池
private readonly ObjectPool<StringBuilder> _stringBuilderPool =
new DefaultObjectPool<StringBuilder>(new StringBuilderPooledObjectPolicy());
public string BuildString()
{
var sb = _stringBuilderPool.Get();
try
{
sb.Append("Hello").Append(" World");
return sb.ToString();
}
finally
{
_stringBuilderPool.Return(sb);
}
}
// 使用Span<T>
public void SpanExample()
{
Span<byte> buffer = stackalloc byte[100];
// 直接在栈上操作,避免堆分配
ReadOnlySpan<char> text = "Hello World".AsSpan();
// 无需创建新的字符串实例
}
}
}
2. 性能优化技巧
C++:
class PerformanceOptimization {
public:
// 内联函数
inline int FastOperation(int x) {
return x * 2;
}
// 常量表达式
constexpr int ConstantCalculation(int x) {
return x * x;
}
// 移动语义
std::vector<int> CreateVector() {
std::vector<int> vec(1000);
return std::move(vec); // 避免复制
}
// 视图和引用
void ProcessLargeData(const std::vector<int>& data) {
// 通过引用避免复制
for (const auto& item : data) {
// 处理数据
}
}
};
C#:
public class PerformanceOptimization
{
// 值类型优化
public struct Point
{
public int X { get; set; }
public int Y { get; set; }
}
// 内存池化
private readonly ArrayPool<byte> _arrayPool = ArrayPool<byte>.Shared;
public async Task ProcessDataAsync()
{
byte[] buffer = _arrayPool.Rent(1024);
try
{
await ProcessBufferAsync(buffer);
}
finally
{
_arrayPool.Return(buffer);
}
}
// 避免装箱
public struct GenericValue<T> where T : struct
{
private readonly T _value;
public GenericValue(T value)
{
_value = value;
}
public override string ToString() => _value.ToString();
}
// 高效字符串处理
public string BuildLargeString()
{
var sb = new StringBuilder();
for (int i = 0; i < 1000; i++)
{
sb.Append($"Item {i},");
}
return sb.ToString();
}
// 异步优化
public async Task<IEnumerable<T>> ProcessItemsAsync<T>(
IEnumerable<T> items,
Func<T, Task> processor)
{
// 批量处理
var batches = items.Chunk(100);
foreach (var batch in batches)
{
var tasks = batch.Select(processor);
await Task.WhenAll(tasks);
}
return items;
}
// SIMD操作
public void SimdOperation()
{
if (Vector.IsHardwareAccelerated)
{
var vector1 = new Vector<float>(new float[] { 1, 2, 3, 4 });
var vector2 = new Vector<float>(new float[] { 5, 6, 7, 8 });
var result = vector1 + vector2;
}
}
}
3. 调试和性能分析
C++:
class Debugging {
public:
void DebugExample() {
#ifdef _DEBUG
std::cout << "Debug mode" << std::endl;
#endif
// 断言
assert(CheckCondition());
// 性能计时
auto start = std::chrono::high_resolution_clock::now();
// 执行代码
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>
(end - start);
}
private:
bool CheckCondition() {
return true;
}
};
C#:
public class Debugging
{
private readonly ILogger<Debugging> _logger;
// 调试辅助方法
[Conditional("DEBUG")]
public void DebugMethod()
{
Debug.WriteLine("Debug information");
Trace.WriteLine("Trace information");
Debug.Assert(CheckCondition(), "Condition failed");
}
// 性能计数器
public async Task MeasurePerformance()
{
using var activity = Activity.StartNew("OperationName");
var sw = Stopwatch.StartNew();
try
{
await PerformOperation();
}
finally
{
sw.Stop();
_logger.LogInformation(
"Operation completed in {Milliseconds}ms",
sw.ElapsedMilliseconds);
}
}
// 诊断工具
public void DiagnosticsExample()
{
// 内存使用
var process = Process.GetCurrentProcess();
var memoryUsed = process.WorkingSet64;
// GC信息
var gcInfo = GC.GetGCMemoryInfo();
var heapSize = gcInfo.HeapSizeBytes;
// 线程信息
var threadCount = Process.GetCurrentProcess().Threads.Count;
}
// 性能计数器
public class PerformanceMonitoring
{
private readonly Metrics _metrics = new();
public async Task MonitorOperation()
{
using (_metrics.MeasureHistogram(
"operation_duration",
"Operation duration in seconds"))
{
await PerformOperation();
}
}
}
}
这些示例展示了C++和C#在性能优化和内存管理方面的不同方法。C++提供了更直接的内存控制,而C#提供了更多的内置工具和自动化机制。
关键区别:
-
C++需要手动管理内存,C#有GC -
C++可以直接操作内存,C#主要通过安全的抽象 -
C++性能优化更依赖于底层控制,C#提供了更多高级工具 -
C#有更完善的性能分析和诊断工具支持
建议C++开发者在转向C#时:
-
了解GC的工作原理 -
熟悉C#的性能优化工具 -
使用适当的内存管理模式 -
利用C#提供的诊断和分析工具
THE END
0
二维码
打赏
海报
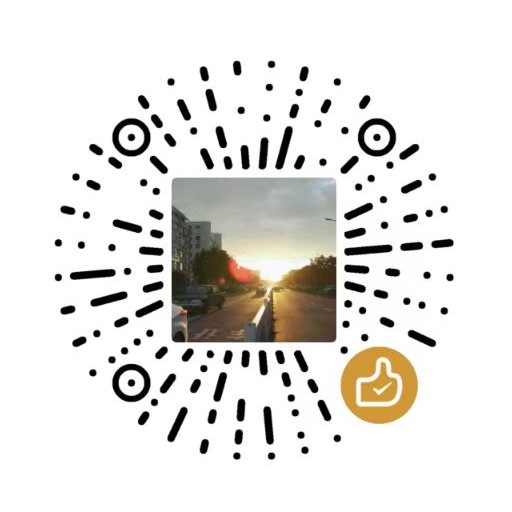
C++到C#核心语法对照指南
C++到C#核心语法对照指南
一、基础语法对比
1. 程序入口点
C++:
int main(int argc, char* argv[]) { std:……
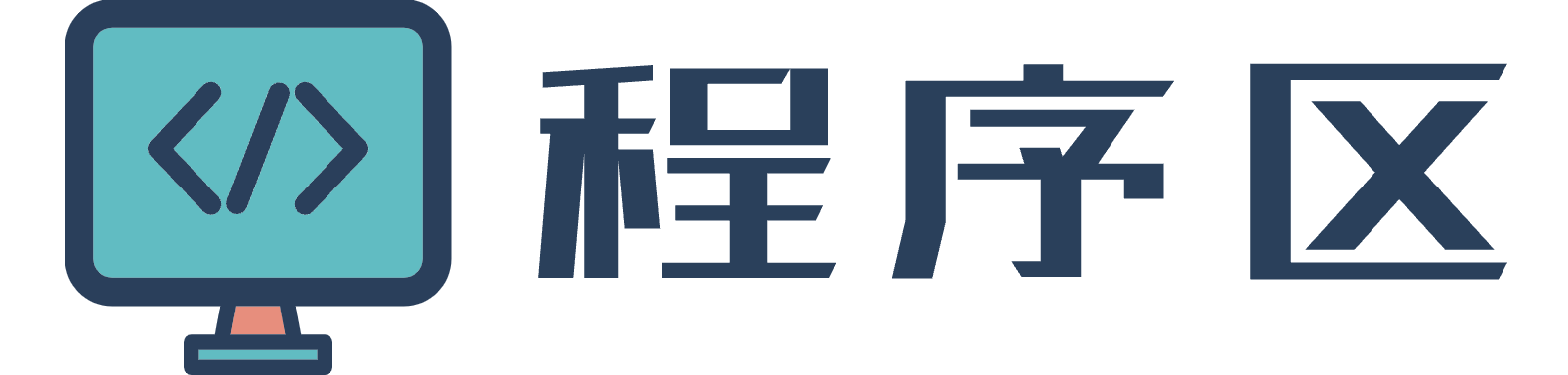